Week 5: Programming Robot Sequences with Blinking LEDs...
Read MoreWeek 6: Controlling Robot Motors with IR Sensor TCRT5000
In Week 6, students will learn to integrate an IR sensor (TCRT5000) with the L298N Motor Driver to control a robot’s movement based on line detection and color detection. The focus will be on programming the robot to move forward when a black line is detected and stop when other colors are detected. This week emphasizes practical applications of sensor integration in robotics.
- Successfully integrate the IR sensor with the L298N Motor Driver and Arduino.
- Demonstrate the ability to program the robot to react to sensor inputs.
- Ensure correct wiring and programming for sensor-based motor control.
- Adhere to safety protocols.
- Collaborate respectfully with peers.
- Review of L298N and Motor Control
- Understanding the TCRT5000 IR Sensor
- Connecting the TCRT5000 to Arduino
- Programming Motor Control with IR Sensor Inputs
- Practice Session
Arduino Board
- Function in Project: Acts as the main microcontroller to run the program and control the LED.
- Other Real-life Uses: Can be used in robotics, home automation, weather stations, and many other DIY electronic projects.
Breadboard
- Function in Project: Provides a platform to build and test circuits without soldering.
- Other Real-life Uses: Useful for prototyping circuits in electronics development and for educational purposes.
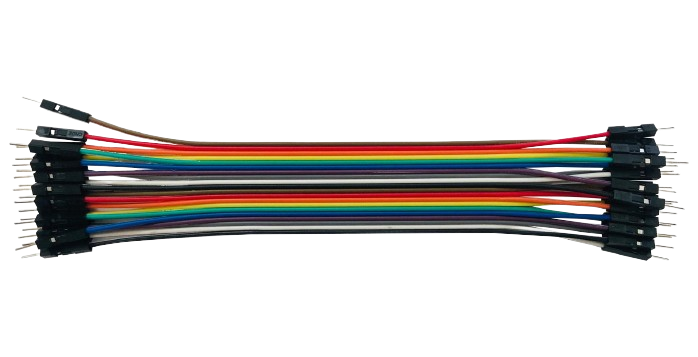
Jumper Wires
- Function in Project: Connect different components on the breadboard and to the Arduino.
- Other Real-life Uses: Used for making temporary connections in testing and prototyping electronic circuits.
L298N Motor Driver
- Function in Project: Controls the speed and direction of the motor.
- Other Real-life Uses: Used in various robotics and motor control applications to drive DC and stepper motors.
Gear Motors
- Function in Project: Converts electrical energy into mechanical movement to drive the robot forward.
- Other Real-life Uses: Used in fans, toys, and various mechanical devices requiring rotary motion.
TCRT5000 IR Sensor
- Function in Project: Detects the presence or absence of an object and sends corresponding data to the Arduino.
- Other Real-life Uses: Used in line-following robots, object detection, and other sensor-based applications.
Review of L298N and Motor Control
- Objective: Recap how to control motors using the L298N Motor Driver.
- Review previous week’s content to ensure students understand motor control basics.
Understanding the TCRT5000 IR Sensor
Learn how the TCRT5000 IR sensor works.
- Function: The TCRT5000 IR sensor emits infrared light and detects reflections to determine the presence of a line or object.
- Detection: It returns different voltage levels based on the amount of reflected light, which can be interpreted as line or color detection.
Connecting the TCRT5000 to Arduino
Wire the TCRT5000 IR sensor to the Arduino.
Steps:
- Power Connections: Connect VCC to 5V and GND to ground.
- Signal Connection: Connect the OUT pin to an Arduino analog or digital input pin (e.g., A0).
Write and upload code to control motors based on IR sensor input.
#include <RoboticCar.h> int EnA = 3; int In1 = 4; int In2 = 5; int EnB = 6; int In3 = 7; int In4 = 8; RoboticCar myrobot(In1, In2, In3, In4, EnA, EnB); int sensorPin = 2; // TCRT5000 sensor connected to digital pin 2 void setup() { pinMode(sensorPin, INPUT); } void loop() { int sensorValue = digitalRead(sensorPin); if (sensorValue == HIGH) { // Adjust based on sensor reading // Black line detected myrobot.forward(200); } else { // No black line detected or other color detected myrobot.stop(); //or myrobot.forward(0); } }
- Objective: Test and adjust the robot’s behavior based on sensor input.
- Activity:
- Place the robot on a line-following track.
- Adjust the sensor threshold and test the robot’s response to line and color detection.
- Experiment with different movements and scenarios.
4WD robot 4
Week 4: Programming 4 Motors with L298N Motor...
Read More4WD robot 3
Week 3: Programming 1 Motor to Drive Forward...
Read More4WD robot 2
Week 2: LED Blink and Patterns Summary In...
Read More