Week 5: Programming Robot Sequences with Blinking LEDs...
Read MoreWeek 5: Programming Robot Sequences with Blinking LEDs
In Week 5, students will program the robot to perform various movement sequences while also controlling LED bulbs to blink in synchronization. This exercise will combine motor control with additional outputs, enhancing the complexity and functionality of their projects.
- Program the robot to move in three different sequences.
- Integrate LED blinking patterns with motor control.
- Demonstrate understanding of complex programming and hardware integration.
- Adhere to safety protocols.
- Collaborate respectfully with peers.
- Review of Motor and LED Control
- Module 1: Robot Movement with Blinking LEDs
- Module 2: Turning Left and Right with Blinking LEDs
- Module 3: Spinning and Stopping with Blinking LEDs
- Module 4: Robot Maneuvers with Blinking LEDs
- Module 5: Coordinated Movements with Blinking LEDs
- Practice Session
Arduino Board
- Function in Project: Acts as the main microcontroller to run the program and control the LED.
- Other Real-life Uses: Can be used in robotics, home automation, weather stations, and many other DIY electronic projects.
Breadboard
- Function in Project: Provides a platform to build and test circuits without soldering.
- Other Real-life Uses: Useful for prototyping circuits in electronics development and for educational purposes.
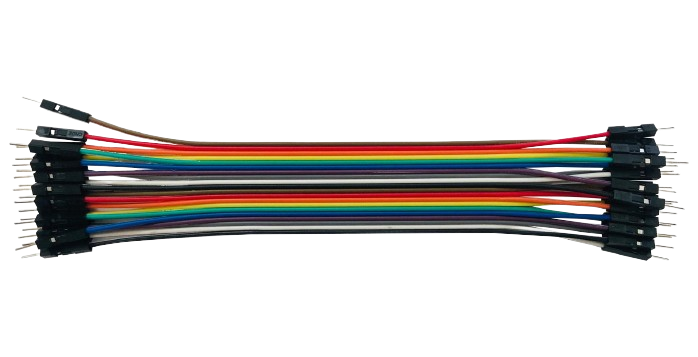
Jumper Wires
- Function in Project: Connect different components on the breadboard and to the Arduino.
- Other Real-life Uses: Used for making temporary connections in testing and prototyping electronic circuits.
L298N Motor Driver
- Function in Project: Controls the speed and direction of the motor.
- Other Real-life Uses: Used in various robotics and motor control applications to drive DC and stepper motors.
Gear Motors
- Function in Project: Converts electrical energy into mechanical movement to drive the robot forward.
- Other Real-life Uses: Used in fans, toys, and various mechanical devices requiring rotary motion.
Objective: Program the robot to move forward and backward while blinking LEDs
.
.
#include <RoboticCar.h> int EnA = 3; int In1 = 4; int In2 = 5; int EnB = 6; int In3 = 7; int In4 = 8; int ledPin = 9; RoboticCar myrobot(In1, In2, In3, In4, EnA, EnB); void setup() { pinMode(ledPin, OUTPUT); } void loop() { digitalWrite(ledPin, HIGH); myrobot.forward(200); delay(1000); digitalWrite(ledPin, LOW); myrobot.stop(); delay(1000); digitalWrite(ledPin, HIGH); myrobot.reverse(200); delay(1000); digitalWrite(ledPin, LOW); myrobot.stop(); delay(1000); }
Objective: Program the robot to turn left and right while blinking LEDs.
#include <RoboticCar.h> int EnA = 3; int In1 = 4; int In2 = 5; int EnB = 6; int In3 = 7; int In4 = 8; int ledPin = 9; RoboticCar myrobot(In1, In2, In3, In4, EnA, EnB); void setup() { pinMode(ledPin, OUTPUT); } void loop() { digitalWrite(ledPin, HIGH); myrobot.left(200); delay(1000); digitalWrite(ledPin, LOW); myrobot.stop(); delay(1000); digitalWrite(ledPin, HIGH); myrobot.right(200); delay(1000); digitalWrite(ledPin, LOW); myrobot.stop(); delay(1000); }
Objective: Program the robot to spin and stop while blinking LEDs.
#include <RoboticCar.h> int EnA = 3; int In1 = 4; int In2 = 5; int EnB = 6; int In3 = 7; int In4 = 8; int ledPin = 9; RoboticCar myrobot(In1, In2, In3, In4, EnA, EnB); void setup() { pinMode(ledPin, OUTPUT); } void loop() { digitalWrite(ledPin, HIGH); myrobot.left(200); delay(500); myrobot.right(200); delay(500); myrobot.stop(); delay(1000); }
Objective: Program the robot for complex maneuvers like zig-zag or circular paths while blinking LEDs.
#include <RoboticCar.h> int EnA = 3; int In1 = 4; int In2 = 5; int EnB = 6; int In3 = 7; int In4 = 8; int ledPin = 9; RoboticCar myrobot(In1, In2, In3, In4, EnA, EnB); void setup() { pinMode(ledPin, OUTPUT); } void loop() { digitalWrite(ledPin, HIGH); myrobot.left(200); delay(500); myrobot.right(200); delay(500); myrobot.forward(200); delay(1000); digitalWrite(ledPin, LOW); myrobot.stop(); delay(1000); }
Objective: Program the robot to perform coordinated movements while blinking LEDs.
#include <RoboticCar.h> int EnA = 3; int In1 = 4; int In2 = 5; int EnB = 6; int In3 = 7; int In4 = 8; int ledPin = 9; RoboticCar myrobot(In1, In2, In3, In4, EnA, EnB); void setup() { pinMode(ledPin, OUTPUT); } void loop() { digitalWrite(ledPin, HIGH); myrobot.left(200); delay(500); myrobot.right(200); delay(500); myrobot.forward(200); delay(1000); digitalWrite(ledPin, LOW); myrobot.stop(); delay(1000); }
4WD robot 4
Week 4: Programming 4 Motors with L298N Motor...
Read More4WD robot 3
Week 3: Programming 1 Motor to Drive Forward...
Read More4WD robot 2
Week 2: LED Blink and Patterns Summary In...
Read More